Harvey Mudd College
Computer Science 60
Spring 1998
Assignments 5 and 6
Assignment 5 due Thurs. 3/5/98, 50 points
Assignment 6 due Thurs. 3/12/98, 50 points
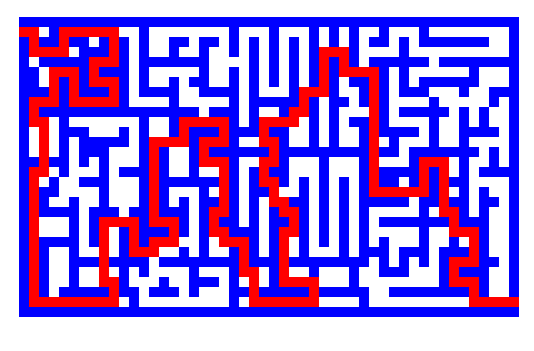
Assignment 5 will provide an opportunity to
create an applet with simple graphics. Assignment 6 extends this
to use a queue (similar to one you constructed in assignment 4)
to perform breadth-first search of a maze. Maze
Maker is a tool for constructing mazes. The user drags the
mouse within the rectangular field to create internal walls, as
shown in blue above. The object of the maze player is to find a
path constructed by the user. Maze Maker is required to display a
minimal-length path through the maze, as shown in red above. In
Assignment 5, your applet need only allow the user to draw a
maze. In assignment 6, path creation is tobe added.
The rules for maze creation (assignment 5) are as follows:
- The drawing field is a grid made of squares of 10 pixels
on a side, 30 by 50 squares total, including outer walls.
- When the user depresses the mouse button, if the cursor
is over a blank square (or a previously-drawn path
square), draw mode is indicated. If the
cursor is over a wall square when the mouse is depressed,
erase mode is indicated.
- In draw mode, a background square over which the
mouse button was depressed, and any squares over which
the mouse is dragged, are changed into wall squares if
they are not wall squares already.
- In erase mode, the wall square over which the
mouse is depressed, and any squares over which the mouse
is dragged, are erased.
- The start and finish squares of the maze are fixed to the
upper left and lower right areas of the maze, as shown in
the drawing. These squares and the outer wall squares are
never modified by the user.
- Add a Clear button which removes
everything which has been drawn.
- On the sample, you will notice a logo when the applet
first opens; this is not required.
The rules for path creation (assignment 6) are as follows:
- The path, if any, is drawn in red, when the mouse button
is released.
- While the user is drawing, the previous path remains on
the screen, except as overwritten by drawing.
- The path must be minimal-length, in the sense that there
is no shorter path from start to finish.
- A path moves only vertically or horizontally from square
to square, not diagonally.
- If there is no path possible, then no red squares are
drawn.
You may want to check out Square.java
(run
the applet) to see how to draw a square and track mouse
events. Use the
method of an off-screen buffer, as discussed in class and shown
in the example, so that your applet does not flicker. This is
mandatory. Applets require an html file. The text for this one is
Square.html.
For the path-finding, breadth-first search will be explained
in the lecture.You may also read about breadth-first
search of a graph in the low-level functional programming
chapter. The concept is the same, but the graph is implicit in
the case of a maze.